A Clear Guide to PHP Email Validation
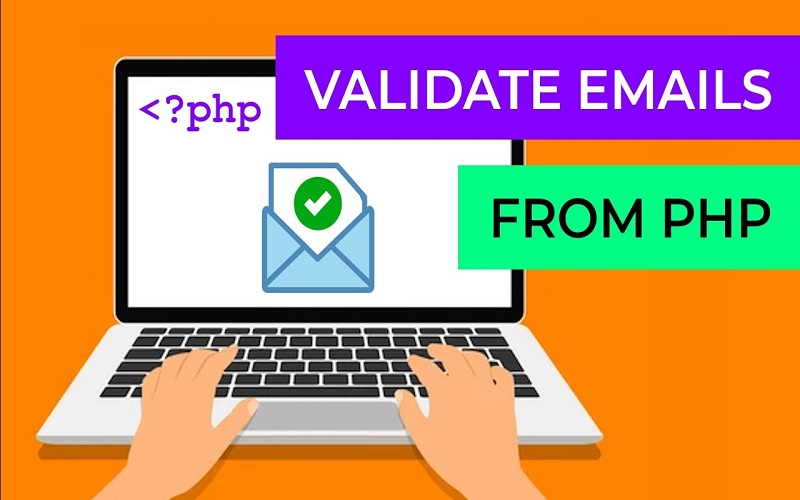
For transactional and marketing communications, validating email addresses is essential to safeguarding the reputation of your email domain. Assuming you have a basic understanding of PHP programming, this guide will assist you in understanding PHP email validation. Learn how to check email validation in PHP
Distinguishing Email Verification From Validation
Email validation verifies the accuracy of an email address’ formatting. Although it’s a step in the verification process, it doesn’t verify that the email address is real. The main topic of this tutorial is PHP server-side validation.
Filter_var() is Used for Email Validation
Using PHP’s `filter_var()` function is an easy method to verify and clean email addresses. It guarantees that the email address is safely stored or processed and formatted correctly.
Here’s an illustration of how to use `filter_var()` to sanitize an email address:
{{{php \?php
“[email protected]”; $userInputEmail =
$saniizedEmail is equal to filter_var(FILTER_SANITIZED_EMAIL, $userInputEmail);
echo “Sanitized: “. $sanitizedEmail;?> {{{ echo “Original: “. $userInputEmail. “
“;
The email address is safe to use once this script eliminates harmful characters.
Using Filter_Validate_EMAIL to Validate Emails
You can use `FILTER_VALIDATE_EMAIL} to validate the email format after sanitizing. This function verifies that the email contains a domain, a @ symbol, and a valid username.
This is how to apply it:
{{{php \?php
“[email protected]” is what $userInputEmail =;
If (filter_var(FILTER_VALIDATE_EMAIL, $userInputEmail)) otherwise { echo “The email address ‘$userInputEmail’ is considered invalid.”; }?> {{{
Regular Expressions for Validating Email Addresses
A versatile method for validating emails with particular needs not addressed by `FILTER_VALIDATE_EMAIL} is to use regular expressions, or regex.
Here’s a simple regex example:
{{{php \?php
Using “[email protected]” as $email
$pattern = ‘/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/’;
Preg_match($pattern, $email) if ( ) otherwise { echo “The email address ‘$email’ is considered invalid.”; }}?> {{{
Depending on your demands, you can modify regex patterns to tighten or loosen validation criteria.
Email Validation using an API
By determining whether an email address is genuine and capable of receiving emails, APIs offer enhanced email validation. They increase accuracy and use less local resources.
Here’s a simple illustration of how to use an API:
{{{php \?php
$email = “[email protected]”; $apiUrl = “https://api.emailvalidator.com/validate?apiKey={$apiKey}&email={$email}”; $apiKey = “YOUR_API_KEY”;
if ($response!== false) {} $data = json_decode($response, true); print_r($data); } else { echo “API request failed.”; }?>
Email Testing using Mailtrap
An email sandbox called Mailtrap is useful for testing and debugging emails in development settings. Mailtrap does not come with email validation, but you can use custom validation scripts in conjunction with it.
This is a unique PHP script that uses an API to validate emails:
{{{php \?php function validEmail($email) $apiUrl = “https://api.emailvalidator.com/validate?apiKey={$apiKey}&email={$email}”; { $apiKey = ‘YOUR_API_KEY’;
File_get_contents($apiUrl) = $response; if ($response!== false) The $data variable is set to json_decode($response, true);
yield false; return $data[‘isValid’];
$email = ‘[email protected]’; if (validateEmail($email)) { ` echo “Email is valid.\n”;
Otherwise, { echo “Email is invalid.\n”; }?> {}}
On a Linux server, you may use a cron job to automate this script:
{{{shell 0 1 * * * /path/to/your/script.php /usr/bin/php
{~